新規作成日 2020-02-15
最終更新日
RichTextBoxの文書を操作する場合、カーソル位置を移動して、カーソル位置を基準に文書を操作する方法があります。RichTextBoxのサンプルコードの中には、カーソル位置を操作する方法を紹介しているものがあります。
参考
WPFで、UIを作成する際、xaml表記を使用すると簡単に表記できます。しかし、データやユーザー操作によって、プログラムの実行中にUIを操作する際には、コードで操作する必要があります。
WPFのRichTextBoxで、カーソルを文書の末尾に移動する
using System;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
namespace RichTextBoxCaretPosition
{
class RichTextBoxCaretPosition : Window
{
[STAThread]
public static void Main()
{
Application app = new Application();
app.Run(new RichTextBoxCaretPosition());
}
public RichTextBoxCaretPosition()
{
StackPanel myStackPanel = new StackPanel();
// ウィンドウの大きさを設定する
Width = 200;
Height = 120;
// 新しいFlowDocumentを作成し、3つの段落を追加します。
FlowDocument flowDoc = new FlowDocument();
// 段落をFlowDocumentに追加します。
flowDoc.Blocks.Add(new Paragraph(new Run("Paragraph 1")));
flowDoc.Blocks.Add(new Paragraph(new Run("Paragraph 2")));
flowDoc.Blocks.Add(new Paragraph(new Run("Paragraph 3")));
// FlowDocumentを、新しいRichTextBoxのコンテンツに設定します。
RichTextBox rtb = new RichTextBox(flowDoc);
myStackPanel.Children.Add(rtb);
this.Content = myStackPanel;
//フォーカスを設定する
rtb.Focus();
// 現在のキャレット位置を取得します。
TextPointer caretPos = rtb.CaretPosition;
// TextPointerを、現在の文書の最後に設定します。
caretPos = caretPos.DocumentEnd;
// 現在の文書の最後に新しいキャレットの位置を指定します。
rtb.CaretPosition = caretPos;
}
}
}
実行結果
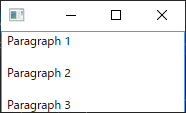
末尾で、カーソルが点滅していることを確認できます。
WPFのRichTextBoxで、カーソルの移動量を設定してカーソルを移動する
using System;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
namespace SetCaretCursorPositionInRichTextBox
{
class SetCaretCursorPositionInRichTextBox : Window
{
[STAThread]
public static void Main()
{
Application app = new Application();
app.Run(new SetCaretCursorPositionInRichTextBox());
}
public SetCaretCursorPositionInRichTextBox()
{
StackPanel myStackPanel = new StackPanel();
// ウィンドウの大きさを設定する
Width = 200;
Height = 200;
// 新しいFlowDocumentを作成し、3つの段落を追加します。
FlowDocument flowDoc = new FlowDocument();
// 段落をFlowDocumentに追加します。
flowDoc.Blocks.Add(new Paragraph(new Run("Paragraph 1")));
flowDoc.Blocks.Add(new Paragraph(new Run("Paragraph 2")));
flowDoc.Blocks.Add(new Paragraph(new Run("Paragraph 3")));
// FlowDocumentを、新しいRichTextBoxのコンテンツに設定します。
RichTextBox rtb = new RichTextBox(flowDoc);
myStackPanel.Children.Add(rtb);
this.Content = myStackPanel;
Button button = new Button() { Content = "Set Cursor Pos" };
myStackPanel.Children.Add(button);
// ボタンのクリックイベント
button.Click += (sender, e) =>
{
//フォーカスを設定する
rtb.Focus();
// 現在のキャレット位置を取得します。
TextPointer caretPos = rtb.CaretPosition;
//移動量を設定する
int displacement = 6;
// 後ろにTextPointer 6移動を設定します
// あなたは、GetPositionAtOffsetを使用して、caretPosを前後に移動し、移動する変位の量を設定できます。:
caretPos = caretPos.GetPositionAtOffset(displacement, LogicalDirection.Backward);
// RichTextBoxに新しいキャレット位置を指定します
// キャレット位置が文書の範囲外だと例外が発生する。
rtb.CaretPosition = caretPos;
};
}
}
}
実行結果
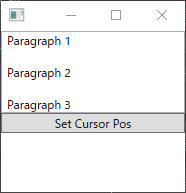
キャレット位置が文書の範囲外だと例外が発生します。「ディバッグなしで実行」する場合は、異常終了します。
