How to: Hit Test Using Geometry as a Parameter
この例は、ヒット・テスト・パラメータとして、Geometryを使用し、ビジュアル・オブジェクト上で、ヒット・テストを、どのように、実行するかを示します。
例
Example
次の例は、HitTestメソッドのGeometryHitTestParametersを使用して、ヒット・テストを、どのように設定するかを示しています。
OnMouseDownメソッドに渡されるPoint値は、ヒット・テストの範囲を拡大するGeometryオブジェクトを作成するために使用されます。
// Respond to the mouse button down event by setting up a hit test results callback.
// ヒット・テスト結果のコールバックを設定することで、マウスの右ボタン・ダウン・イベントに応答します。
private void OnMouseDown(object sender, MouseButtonEventArgs e)
{
// Retrieve the coordinate of the mouse position.
// マウス位置の座標を取り出します。
Point pt = e.GetPosition((UIElement)sender);
// Expand the hit test area by creating a geometry centered on the hit test point.
// ヒット・テストの点を中心とするジオメトリを作成して、ヒット・テスト領域を拡張します。
EllipseGeometry expandedHitTestArea = new EllipseGeometry(pt, 10.0, 10.0);
// Clear the contents of the list used for hit test results.
// ヒット・テスト結果のために使用されるリストのコンテンツをクリアします。
hitResultsList.Clear();
// Set up a callback to receive the hit test result enumeration.
// ヒット・テスト結果の列挙体を受け取るために、コールバックを設定します。
VisualTreeHelper.HitTest(myControl, null,
new HitTestResultCallback(MyHitTestResultCallback),
new GeometryHitTestParameters(expandedHitTestArea));
// Perform actions on the hit test results list.
// ヒット・テスト結果のリストで、動作を実行します。
if (hitResultsList.Count > 0)
{
ProcessHitTestResultsList();
}
}
GeometryHitTestResultのIntersectionDetailプロパティは、ヒット・テスト・パラメータとして、Geometryを使用する、ヒット・テストの結果に関する情報を提供します。次の図は、ヒット・テスト・ジオメトリー(青い円)とターゲットのビジュアル・オブジェクト(赤い正方形)のレンダリングされたコンテンツの間の関係を示しています。
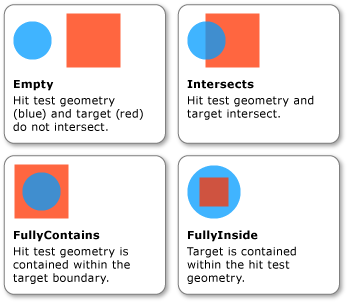
- Empty
ヒット・テスト・ジオメトリー(青い)とターゲット(赤い)は、交差しません。
- Instersects
ヒット・テスト・ジオメトリーとターゲットが、交差します。
- FullyContains
ヒット・テスト・ジオメトリーは、ターゲット境界内に含まれています。
- FullyInside
ターゲットは、ヒット・テスト・ジオメトリー内に含まれます。
次の例は、Geometryが、ヒット・テスト・パラメータとして使用されるとき、ヒット・テスト・コールバックを、どのように、実装するかを示しています。結果パラメータは、IntersectionDetailプロパティの値を取り出すために、GeometryHitTestResultにキャストされます。
Geometryのヒット・テスト・パラメータが、fully、あるいは、ヒット・テスト・ターゲットのレンダリングされたコンテンツの範囲内に部分的に、含まれている場合、プロパティ値は、あなたが、決定できます。この場合、サンプル・コードは、ヒット・テストの結果をビジュアルのリストに追加するだけです。ターゲットの境界の中に、完全に含まれています。
// Return the result of the hit test to the callback.
// コールバックに、ヒット・テストの結果を返します。
public HitTestResultBehavior MyHitTestResultCallback(HitTestResult result)
{
// Retrieve the results of the hit test.
// ヒット・テストの結果を取り出します。
IntersectionDetail intersectionDetail = ((GeometryHitTestResult)result).IntersectionDetail;
switch (intersectionDetail)
{
case IntersectionDetail.FullyContains:
// Add the hit test result to the list that will be processed after the enumeration.
// 列挙の後で処理される、ヒット・テストの結果を、リストに追加します。
hitResultsList.Add(result.VisualHit);
return HitTestResultBehavior.Continue;
case IntersectionDetail.Intersects:
// Set the behavior to return visuals at all z-order levels.
// すべてのz-order・レベルで、ビジュアルを返すように、動作を設定します。
return HitTestResultBehavior.Continue;
case IntersectionDetail.FullyInside:
// Set the behavior to return visuals at all z-order levels.
// すべてのz-order・レベルで、ビジュアルを返すように、動作を設定します。
return HitTestResultBehavior.Continue;
default:
return HitTestResultBehavior.Stop;
}
}
交差の詳細が、Emptyであるとき、HitTestResultコールバックは、呼び出さないでください。
こちらもご覧ください
See also
