続いて、ファイル・ツールバーを追加します。
RichTextBox内のテキストを保存、読み込み、印刷するためには、その動作を指定するC#コードを記述する必要があります。
記述するコードを作成するために、動作を検証するためのプログラムを作成します。
WPFのRichTextBoxに、ファイルと編集ツールバーを設置する
複数のツールバーを使用するときは、ToolBarTrayを使用することをお勧めします。
動作確認をするために、新たに、ファイルツールバーのみを設置したRichTextBoxを持つ、WPFアプリケーションを作成します。
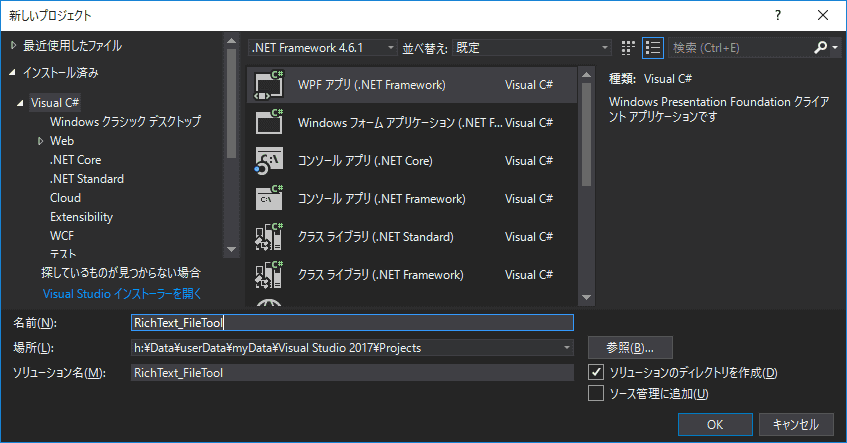
ファイルから、新規作成、プロジェクトを選択し、新しいプロジェクトを作成します。
xamlコード「MainWindow.xaml」にコードを追加して、次のように変更します。
<Window x:Class="RichText_FileTool.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:RichText_FileTool"
mc:Ignorable="d"
Title="MainWindow" Height="250" Width="325">
<DockPanel Name="mainPanel">
<ToolBarTray Name="mainToolbarTray" DockPanel.Dock="Top">
<ToolBar Name="FileToolBar">
<!--ファイルを開く-->
<Button Click="LoadRTBContent" ToolTip="Open">
<Image Source="Images\fileopen.png"></Image>
</Button>
<!--ファイルを保存する-->
<Button Click="SaveRTBContent" ToolTip="Save">
<Image Source="Images\filesave.png"></Image>
</Button>
<!--印刷する-->
<Button Click="PrintRTBContent" ToolTip="Save">
<Image Source="Images\print.png"></Image>
</Button>
</ToolBar>
</ToolBarTray>
<!-- By default pressing tab moves focus to the next control.
既定では、タブを押すと、フォーカスを次のコントロールへ移動します。
Setting AcceptsTab to true allows the RichTextBox to accept tab characters.
AcceptsTab設定をtrueにすると、RichTextBoxは、タブ文字を受け取ることができます。-->
<RichTextBox Name="RTB" AcceptsTab="True"></RichTextBox>
</DockPanel>
</Window>
Imagesフォルダを作成して、アイコンリソースを追加します。
「MainWindows.xaml.cs」に、ツールボタンのイベントとその内容を記述します。
using System;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Media;
using System.IO;
namespace RichText_FileTool
{
/// <summary>
/// MainWindow.xaml の相互作用ロジック
/// </summary>
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
// Handle "Save RichTextBox Content" button click.
// 「RichTextBoxの内容を保存」ボタンのクリックを処理します。
void SaveRTBContent(Object sender, RoutedEventArgs args)
{
// Send an arbitrary URL and file name string specifying
// the location to save the XAML in.
// XAMLを中に保存するための位置を指定する、任意のURLとファイル名の文字列を送ります。
SaveXamlPackage("d:\\test.xaml"); // パス付の保存ファイル名
}
// Handle "Load RichTextBox Content" button click.
// 「RichTextBoxの内容の読込」ボタンのクリックを処理します。
void LoadRTBContent(Object sender, RoutedEventArgs args)
{
// Send URL string specifying what file to retrieve XAML
// from to load into the RichTextBox.
// RichTextBoxに読み込むために、どんなファイルもXAMLを取得するために、指定するURL文字列を送ります。
LoadXamlPackage("d:\\test.xaml"); // パス付の読込ファイル名
}
// Handle "Print RichTextBox Content" button click.
// 「RichTextBoxの内容の印刷」ボタンのクリックを処理します。
void PrintRTBContent(Object sender, RoutedEventArgs args)
{
PrintCommand();
}
// Save XAML in RichTextBox to a file specified by _fileName
// _fileNameで指定するファイルを、RichTextBoxでXAMLを保存します。
void SaveXamlPackage(string _fileName)
{
TextRange range;
FileStream fStream;
range = new TextRange(RTB.Document.ContentStart, mainRTB.Document.ContentEnd);
fStream = new FileStream(_fileName, FileMode.Create);
range.Save(fStream, DataFormats.XamlPackage);
fStream.Close();
}
// Load XAML into RichTextBox from a file specified by _fileName
// _fileNameで指定されたファイルから、RichTextBoxにXAMLを読み込みます
void LoadXamlPackage(string _fileName)
{
TextRange range;
FileStream fStream;
if (File.Exists(_fileName))
{
range = new TextRange(RTB.Document.ContentStart, mainRTB.Document.ContentEnd);
fStream = new FileStream(_fileName, FileMode.OpenOrCreate);
range.Load(fStream, DataFormats.XamlPackage);
fStream.Close();
}
}
// Print RichTextBox content
// RichTextBox内容を印刷する
private void PrintCommand()
{
PrintDialog pd = new PrintDialog();
if ((pd.ShowDialog() == true))
{
// use either one of the below
// 以下のどちらかを使用してください。
pd.PrintVisual(RTB as Visual, "printing as visual");
pd.PrintDocument((((IDocumentPaginatorSource)mainRTB.Document).DocumentPaginator), "printing as paginator");
}
}
}
}
開始をクリックします。
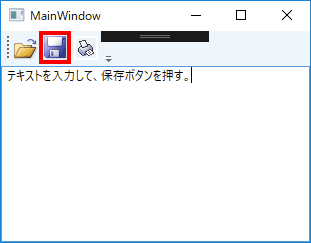
ウィンドウが表示されます。RichTextBoxにテキストを入力し、保存ボタンを押します。Dドライブ直下に、 test.xamlという名前で、RichTextBoxにテキストの内容が保存されます。
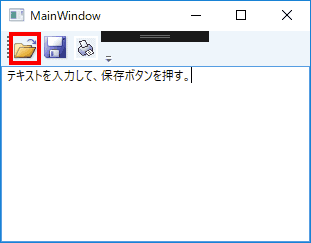
入力したテキストを削除して、読み込みボタンを押すと、保存したテキストが読み込まれます。
保存と読み込みが実装できました。
ダイアログ・ボックスを追加する
ファイル名をコード内に記述するとファイルが選択できません。それでは、使いにくいので、ファイルを選択できるようにします。C#では、ダイアログボックスと呼ばれる選択を支援するコンポーネントが用意されています。読み込み、保存、印刷の3つのダイアログボックスが利用できます。
それでは、実装してみましょう。
「MainWindows.xaml.cs」に、ツールボタンのイベントとその内容を記述します。
using System;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Media;
using System.IO; // ファイルの読み書きに必要
using Microsoft.Win32; // オープンファイル・ダイアログボックスで使用する。
namespace RichText_FileTool
{
/// <summary>
/// MainWindow.xaml の相互作用ロジック
/// </summary>
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
private void SaveRTBContent(object sender, RoutedEventArgs e)
{
// 「RichTextBoxの内容を保存」ボタンのクリックイベントを処理します。
SaveXamlPackage();
}
private void LoadRTBContent(object sender, RoutedEventArgs e)
{
// 「RichTextBoxの内容の読込」ボタンのクリックイベントを処理します。
LoadXamlPackage();
}
private void PrintRTBContent(object sender, RoutedEventArgs e)
{
// 「RichTextBoxの内容の印刷」ボタンのクリックイベントを処理します。
PrintCommand();
}
void SaveXamlPackage()
{
// ファイル名をダイアログで指定して保存します。
// ファイル名を指定します。
// Configure save file dialog box セーブ・ファイル・ダイアログボックスを設定します。
SaveFileDialog dlg = new SaveFileDialog();
dlg.FileName = "Document"; // Default file name 既定のファイル名
dlg.DefaultExt = ".xaml"; // Default file extension 既定のファイル拡張子
dlg.Filter = "RichText documents (.xaml)|*.xaml";
// Filter files by extension 拡張機能によってファイルにフィルターをかけます。
// Show save file dialog box 既定のファイル拡張子
Nullable<bool> result = dlg.ShowDialog();
// Process save file dialog box results
// セーブ・ファイル・ダイアログボックスを表示します。
if (result == true)
{
// ファイルを保存します。
TextRange range;
FileStream fStream;
range = new TextRange(RTB.Document.ContentStart, RTB.Document.ContentEnd);
fStream = new FileStream(dlg.FileName, FileMode.Create);
range.Save(fStream, DataFormats.XamlPackage);
fStream.Close();
}
}
void LoadXamlPackage()
{
// ファイル名を指定して読み込みます。
// ファイル名を指定します。
// Configure open file dialog box オープンファイル・ダイアログボックスを設定します。
OpenFileDialog dlg = new OpenFileDialog();
dlg.FileName = "Document"; // Default file name 既定のファイル名
dlg.DefaultExt = ".xaml"; // Default file extension 既定のファイル拡張子
dlg.Filter = "RichText documents (.xaml)|*.xaml";
// Filter files by extension 拡張機能によってファイルにフィルターをかけます。
// Show open file dialog box オープンファイル・ダイアログボックスを表示します。
bool? result = dlg.ShowDialog();
// Process open file dialog box results オープンファイル・ダイアログボックスの結果を処理します。
if (result == true)
{
// ファイルを読み込みます。
TextRange range;
FileStream fStream;
if (File.Exists(dlg.FileName))
{
range = new TextRange(RTB.Document.ContentStart, RTB.Document.ContentEnd);
fStream = new FileStream(dlg.FileName, FileMode.OpenOrCreate);
range.Load(fStream, DataFormats.XamlPackage);
fStream.Close();
}
}
}
// Print RichTextBox content
// RichTextBox内容を印刷する
private void PrintCommand()
{
PrintDialog pd = new PrintDialog();
if ((pd.ShowDialog() == true))
{
// use either one of the below
// 以下のどちらかを使用してください。
pd.PrintVisual(RTB as Visual, "printing as visual");
pd.PrintDocument((((IDocumentPaginatorSource)RTB.Document).DocumentPaginator), "printing as paginator");
}
}
}
}
ダイアログボックスが実装できました。
